Part 2: Why smart traders use volatility structure to maximize profits
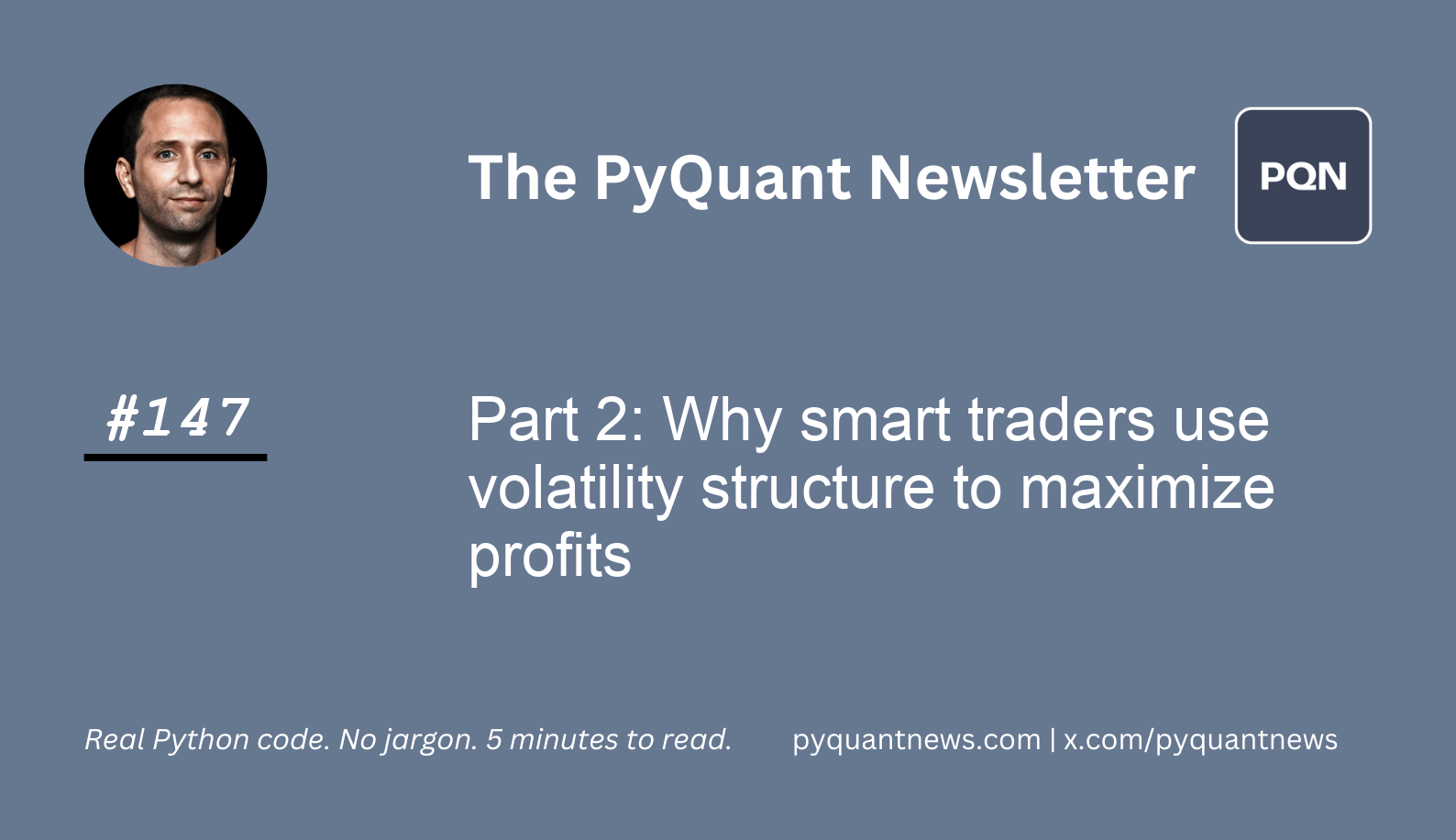
Part 2: Why smart traders use volatility structure to maximize profits
In last week’s newsletter, we looked at how options traders use volatility skew to build great trades.
Today, we’ll explore a related concept called volatility term structure.
The term structure is similar to skew except it plots implied volatility over a series of expirations (for a given strike).
In today’s newsletter, you’ll get Python code to build volatility term structure for call options.
Let’s go!
Part 2: Why smart traders use volatility structure to maximize profits
Volatility term structure sheds light on how implied volatility changes across different option expiration dates. The structure is important for traders who aim to understand future market volatility.
In practice, traders use volatility term structures to select appropriate strategies for current market sentiment. They may choose calendar spreads or straddles based on upward or downward sloping structures.
Professionals analyze volatility term structures to devise sophisticated trading strategies and hedge against potential risks. They utilize these insights to predict market movements and adjust their portfolios accordingly. Having a deep understanding of this concept allows them to exploit opportunities and manage risks efficiently.
Let's see how it works with Python.
Imports and set up
These libraries help us fetch financial data, manipulate it, and create visualizations.
1import numpy as np
2import pandas as pd
3from pandas.tseries.offsets import WeekOfMonth
4import yfinance as yf
5from matplotlib import pyplot as plt
We'll gather option data for a specific stock ticker and organize it into a dataframe.
1ticker = "SPY"
2stock = yf.Ticker(ticker)
3expirations = stock.options
4
5all_options = []
6for dt in expirations:
7 options = stock.option_chain(dt)
8 calls = options.calls
9 calls['expirationDate'] = dt
10 all_options.append(calls)
11
12options_df = pd.concat(all_options)
13options_df['daysToExpiration'] = (pd.to_datetime(options_df['expirationDate']) - pd.Timestamp.now()).dt.days
We start by defining our stock ticker as "SPY" and use yfinance to fetch its data. We then loop through all available expiration dates, collecting call option data for each. We combine this data into a single dataframe and calculate the days to expiration for each option.
Filter and clean the options data
Now we'll filter our options to a specific strike price and clean up the data.
1strike = 590
2filtered_options = options_df[options_df['strike'] == strike]
3
4def clean(df):
5 cols = ["daysToExpiration", "impliedVolatility"]
6 return (
7 df[cols]
8 .dropna(subset=["impliedVolatility"])
9 .drop_duplicates("daysToExpiration")
10 .sort_values("daysToExpiration")
11 .set_index("daysToExpiration")
12 )
13
14def build_iv_grid(df):
15 df_iv = clean(df)
16 df_iv = df_iv.where(df_iv >= 0.01, np.nan)
17 df_iv = df_iv[df_iv.index > 30]
18 expiry_grid = np.arange(df_iv.index.min(), df_iv.index.max() + 1, 1.0)
19 return (
20 df_iv.reindex(expiry_grid)
21 .interpolate(method="linear")
22 .rename_axis("daysToExpiration")
23 ).dropna()
24
25call_structure = build_iv_grid(options_df)
We filter our options to focus on a specific strike price. The clean function keeps only the days to expiration and implied volatility columns, removing any duplicates or missing data. The build_iv_grid function further processes this data, creating a grid of implied volatilities across different expiration dates.
Visualize the term structure
Finally, we'll create a plot to visualize the implied volatility term structure.
1plt.plot(call_structure.index, call_structure.impliedVolatility)
2plt.xlabel('Days to Expiration')
3plt.ylabel('Implied Volatility')
4plt.title(f'Implied Volatility Term Structure for {ticker} (Strike: {strike})')
5plt.grid(True)
6plt.show()
We use matplotlib to create a line plot of our implied volatility data.
.png)
The x-axis shows the days to expiration, while the y-axis shows the implied volatility. We add labels to our axes and a title that includes our stock ticker and strike price.
The term structure shows a steeply declining trend, characteristic of a market expecting heightened short-term uncertainty that gradually diminishes in the long-term.
The unevenness or "unsmooth" nature of the curve results from low liquidity, wide bid-ask spreads, or market mispricing in less actively traded, weekly-expiring options.
Given this structure, one might consider implementing calendar spreads—selling high implied volatility short-term options and buying relatively cheaper long-term options to capitalize on volatility convergence.
Your next steps
In today’s example, we take a shortcut by using Yahoo Finance’s calculation of implied volatility. Most professionals will compute their own. In the meantime, experiment with different underlying stocks or different strikes. See how the term structure changes based on the strike of the option.
